Stencilでビットコイン価格表示アプリ(Progressive Web Apps)を写経してみる(1)レイアウトのレンダリング
前回は、localStorage利用のTodoアプリを途中まで作成しました。
とりあえず、別のStencilアプリを写経して『ビットコイン価格表示アプリ』を作成してみたいと思います。
https://www.joshmorony.com/building-a-pwa-with-stencil-rendering-layouts/
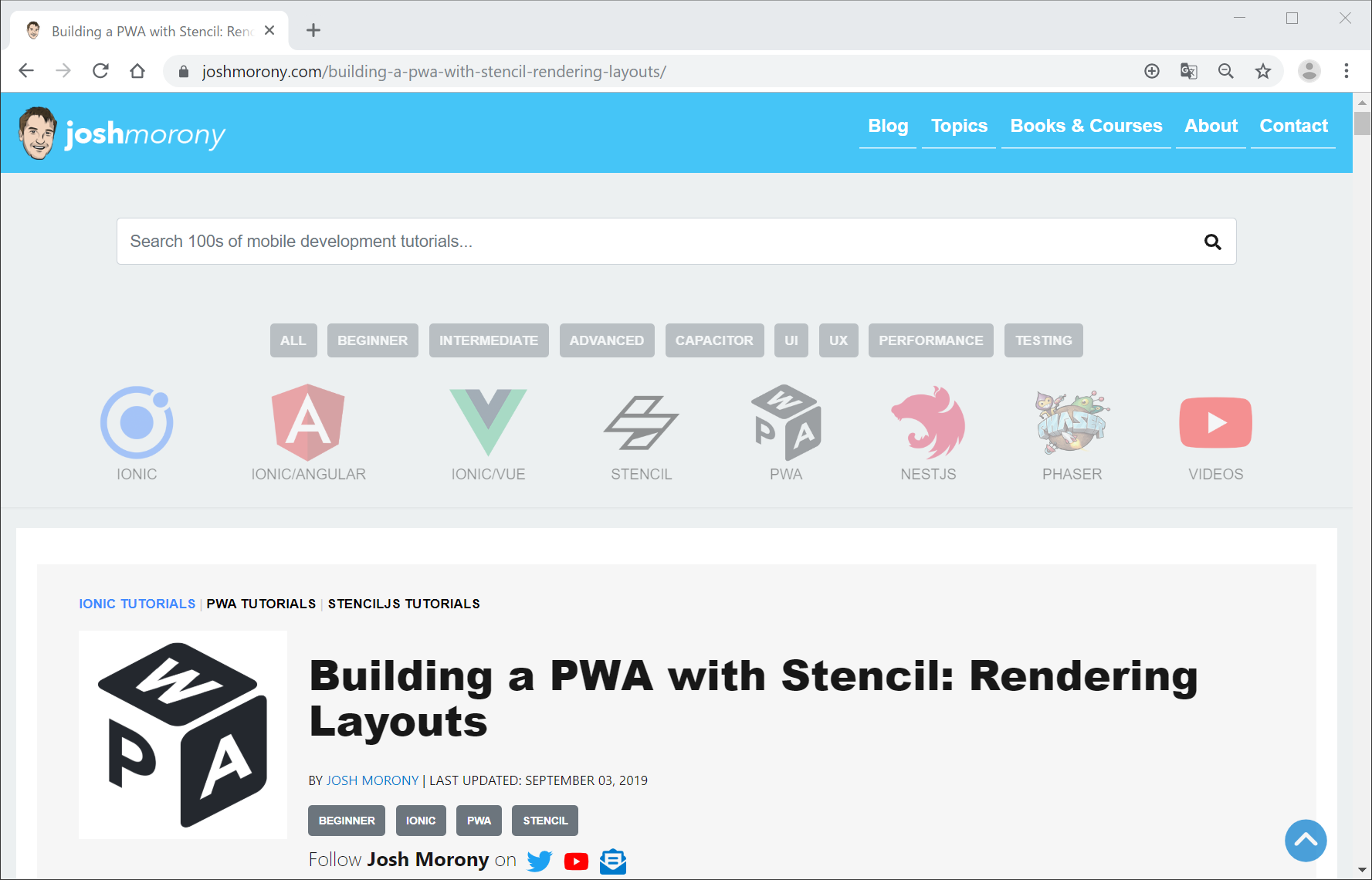
Stencilでビットコイン価格表示アプリ目次
- Stencilでビットコイン価格表示アプリ(Progressive Web Apps)を写経してみる(1)レイアウトのレンダリング
- Stencilでビットコイン価格表示アプリ(Progressive Web Apps)を写経してみる(2)ルーティングとフォーム
- Stencilでビットコイン価格表示アプリ(Progressive Web Apps)を写経してみる(3)ストレージとサービス
ソースコードとDEMOサイト
ソースコード
https://github.com/adash333/stencil-fetch-pwa/tree/a97fa27ca1c7e0e5e3b696788b52ad591562d406
DEMOサイト
開発環境
Windows 10 Pro (1803)
VisualStudioCode 1.37.1
git version 2.20.1.windows.1
nvm-windows 1.1.7
node 12.2.0
npm 6.9.0
yarn 1.16.0
新規Stencilアプリ作成
C:/stencil/ フォルダ内に、stencil-fetch-pwa/ という名前のアプリを作成することにします。
C:/stencil/ フォルダを右クリックして、『Open with Code』をクリックして、VisualStudioCodeで開き、Ctrl+@でターミナル画面を出します。次に、以下を入力して新規stencilアプリを作成します。
npm init stencil
// ionic-pwaを選択して、Enterを押す
// Project name は stencil-fetch-pwa と入力して、Enterを押す
// Confirm? はそのままEnterを押す
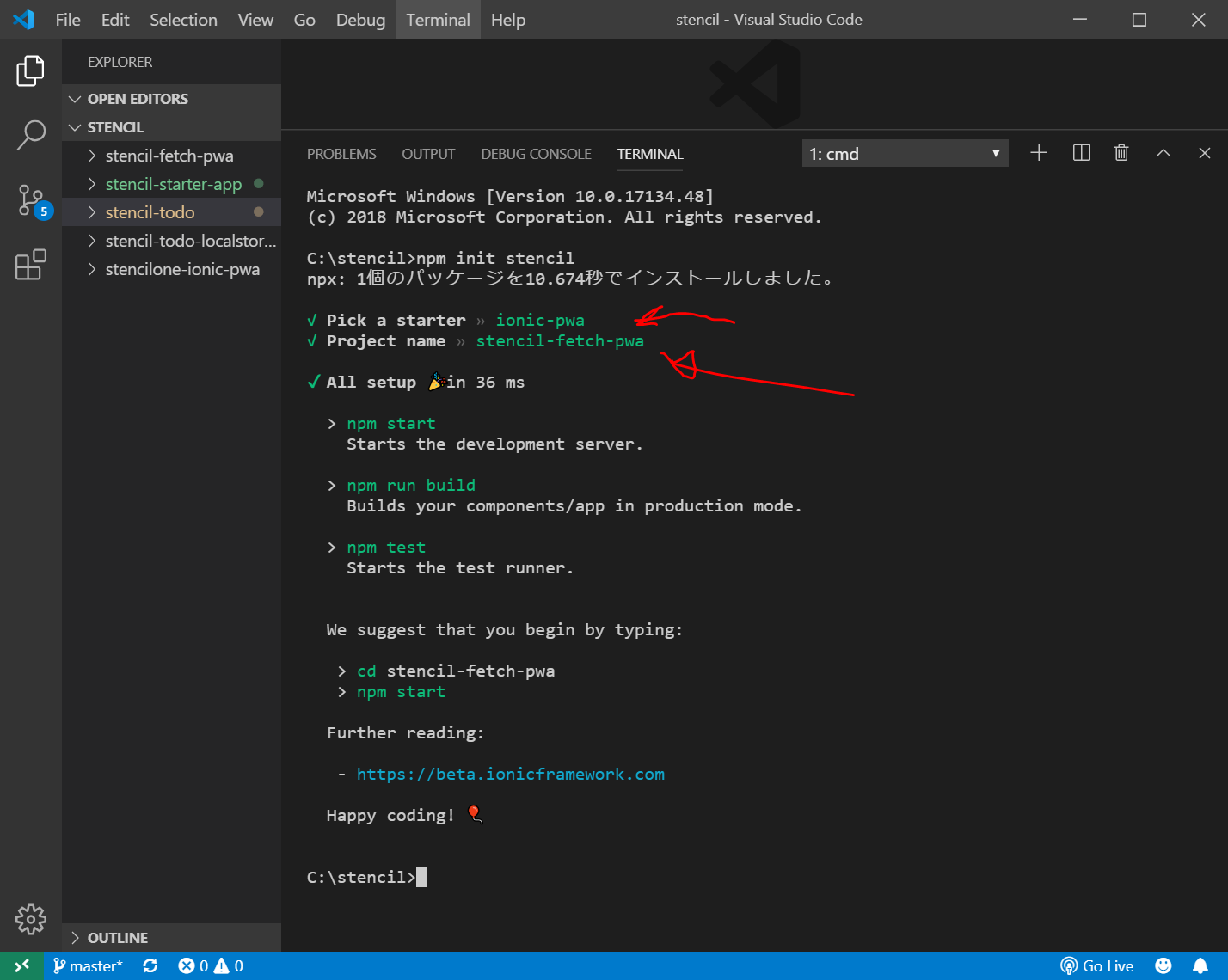
次に、以下を入力して、開発サーバを起動してみます。
(”npm install”が必要です。)
cd stencil-fetch-pwa
npm install
npm start
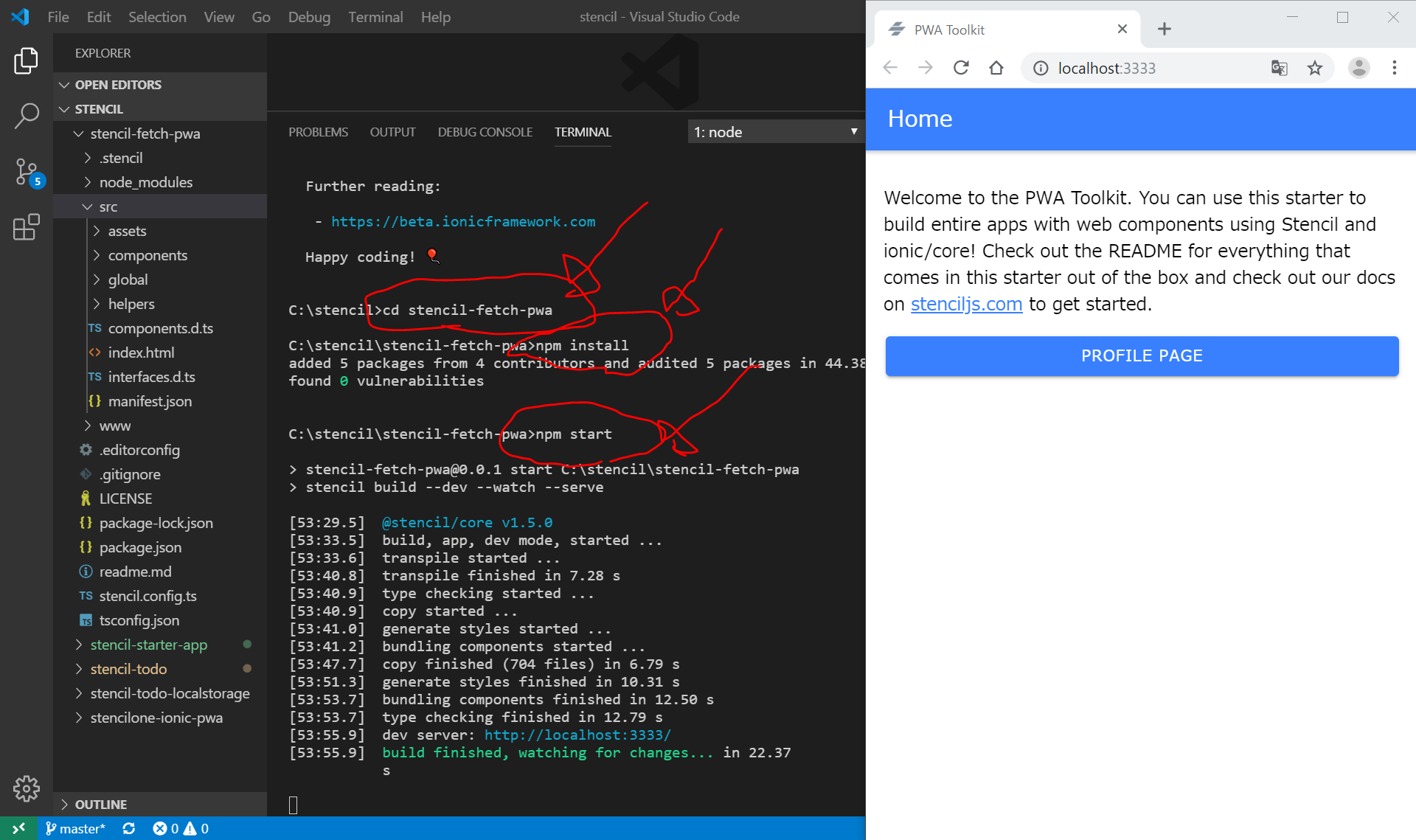
開発サーバを停止するときは、ターミナル画面で、”Ctrl+C” => y + Enter で停止できます。
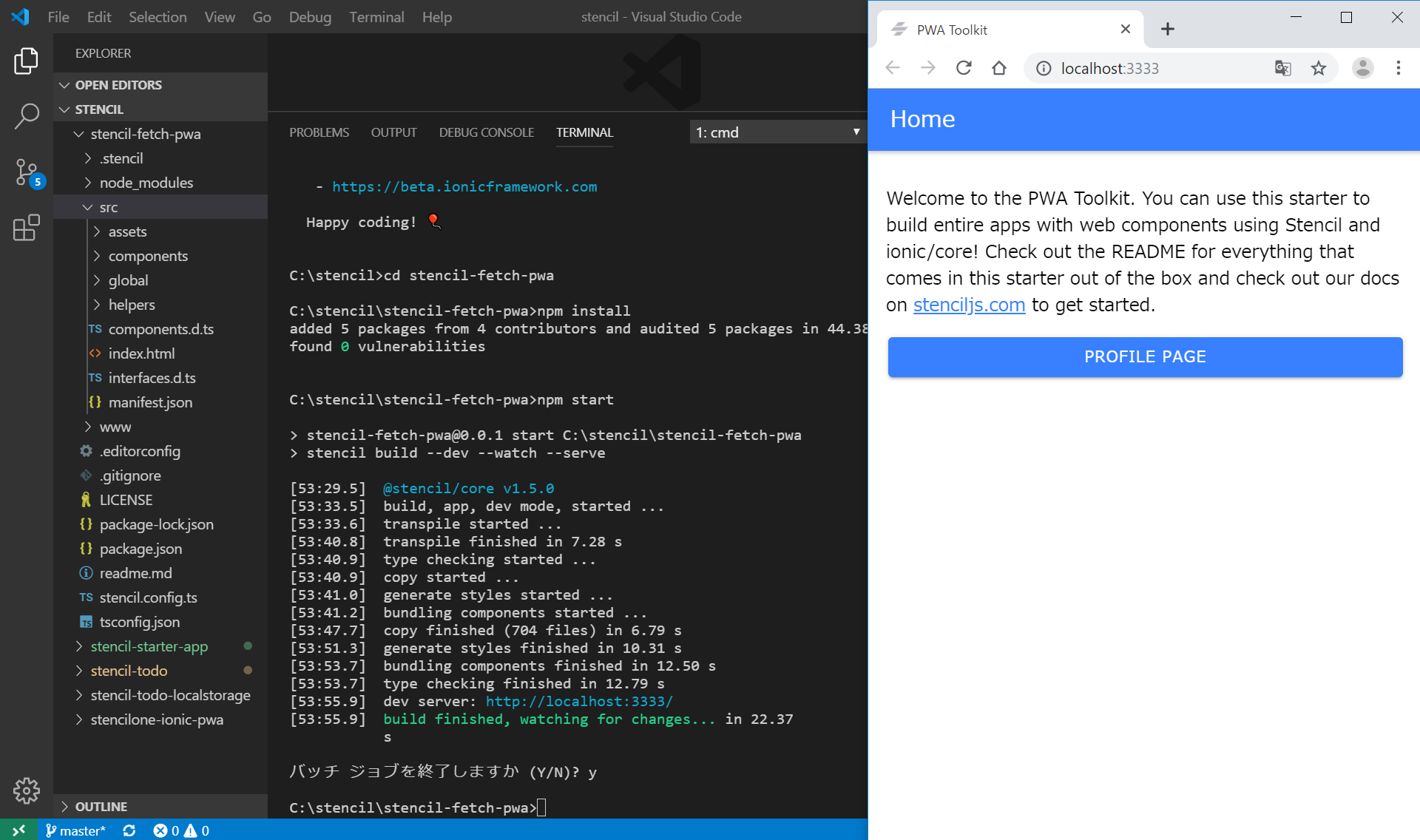
app-home.tsxの編集
src/components/app-home/app-home.tsx を、以下のように変更します。
import { Component, h } from '@stencil/core';
@Component({
tag: 'app-home',
styleUrl: 'app-home.css'
})
export class AppHome {
render() {
return [
<ion-header>
<ion-toolbar color="primary">
<ion-title>BitCoin PWA</ion-title>
</ion-toolbar>
</ion-header>,
<ion-content>
<ion-list lines="none"></ion-list>
</ion-content>,
<ion-footer>
<ion-toolbar>
<p>
<strong>Disclaimer:</strong> Do not use this application to make investment decisions.
Displayed prices may not reflect actual prices.
</p>
</ion-toolbar>
</ion-footer>
];
}
}
(変更前)
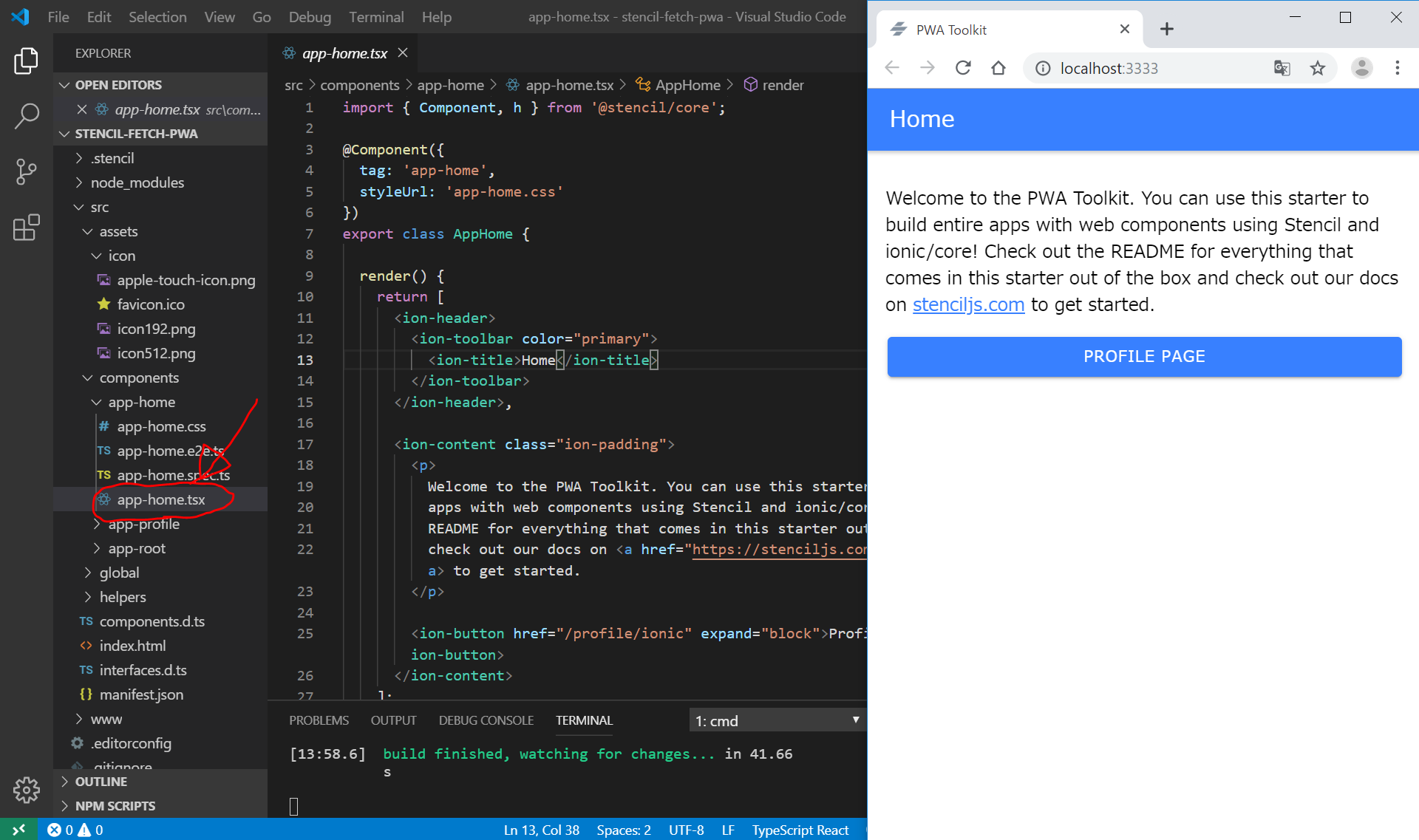
(変更後)
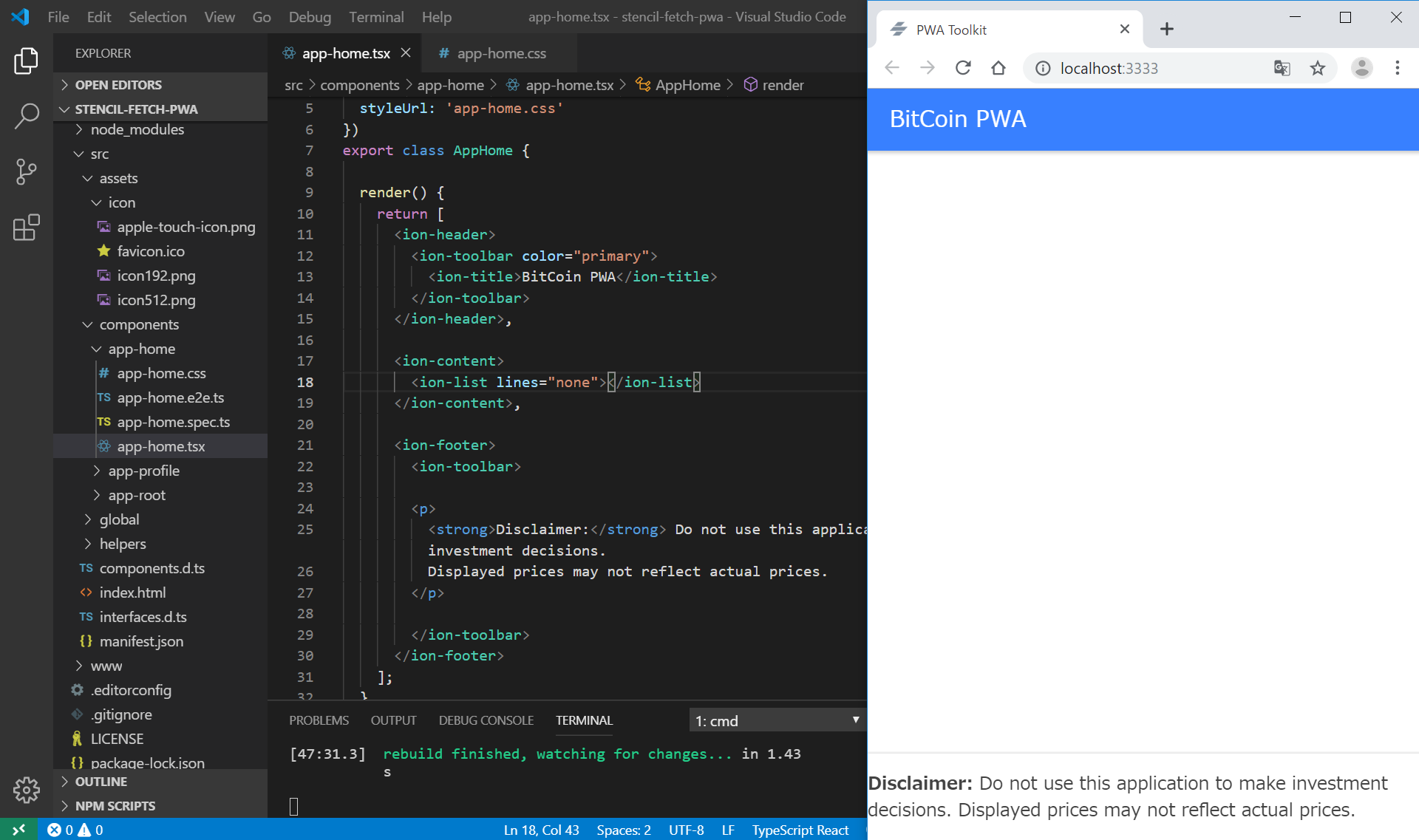
フッターの文字がのpaddingなどは、app-home.css で設定します。
src/components/app-home/app-home.css
ion-footer {
padding: 10px;
font-size: 0.7em;
color: #474747;
background-color: #f6f6f6;
}
ion-item-sliding {
margin-top: 10px;
}
ion-item {
background-color: #f6f6f6;
text-transform: uppercase;
padding: 10px;
}
.amount {
font-size: 0.7em;
}
.value {
margin-top: 10px;
font-size: 1.2em;
color: #32db64;
}
(変更後)
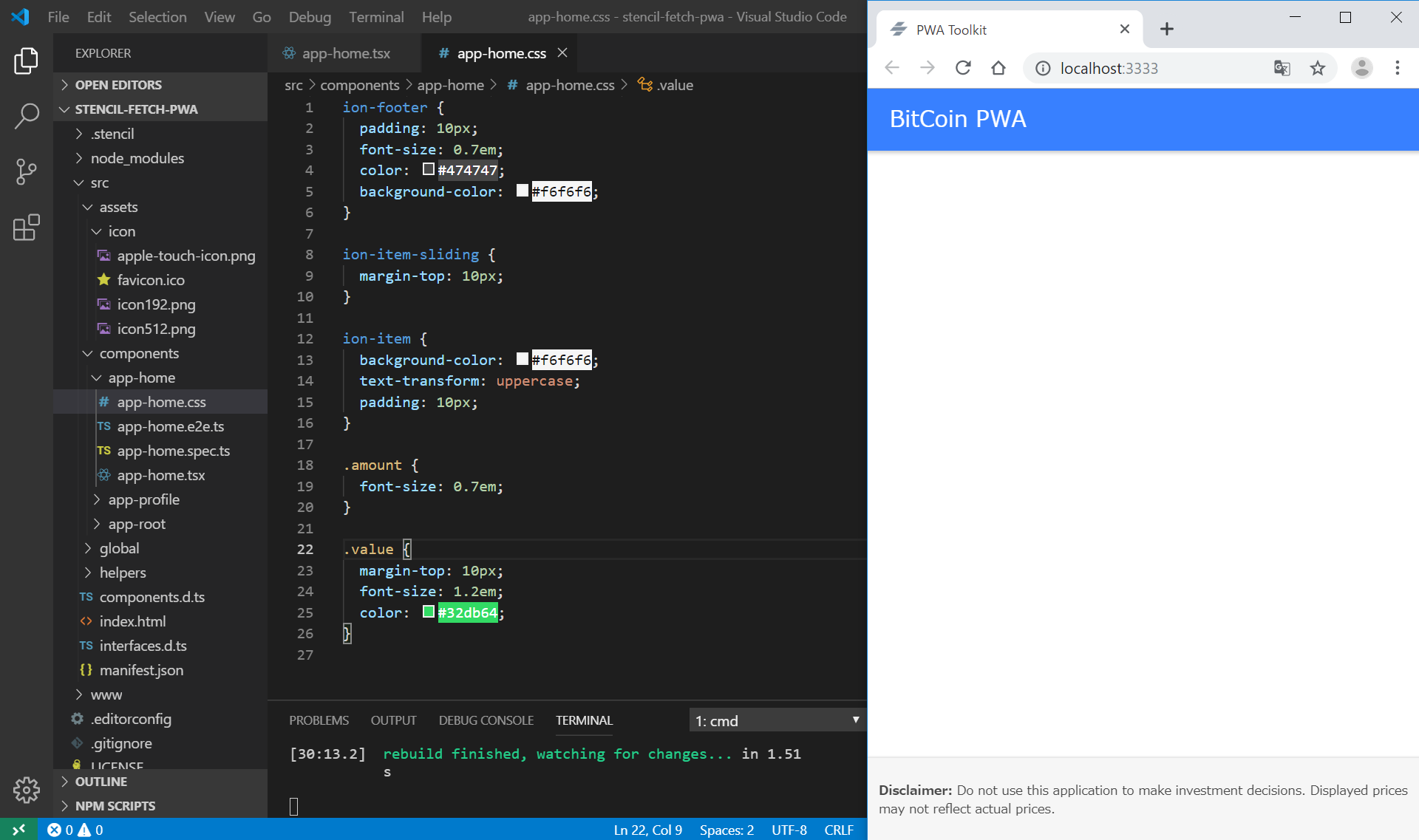
interfaceの定義とcomponentWillLoad()での疑似データの投入
app-home.tsx の上の方に、以下を追加します。
import { Component, State, h } from '@stencil/core';
// interfaceで連想配列Holdingの中身の型の定義を行う
// value? は値が存在しなくてもよいという意味?
interface Holding {
crypto: string;
currency: string;
amount: number;
value?: number;
}
@Component({
tag: 'app-home',
styleUrl: 'app-home.css'
})
export class AppHome {
@State() holdings: Holding[] = [];
componentWillLoad() {
// ダミーデータを投入、後で、http通信でデータを取り込む
this.holdings = [
{
crypto: "BTC",
currency: "USD",
amount: 0.1,
value: 11488.32383
},
{
crypto: "ETH",
currency: "USD",
amount: 2,
value: 1032.23421
},
{
crypto: "BTC",
currency: "JPY",
amount: 4,
value: 103555.223423
}
]
}
(続く...)
(変更後) (画面は変化なし)
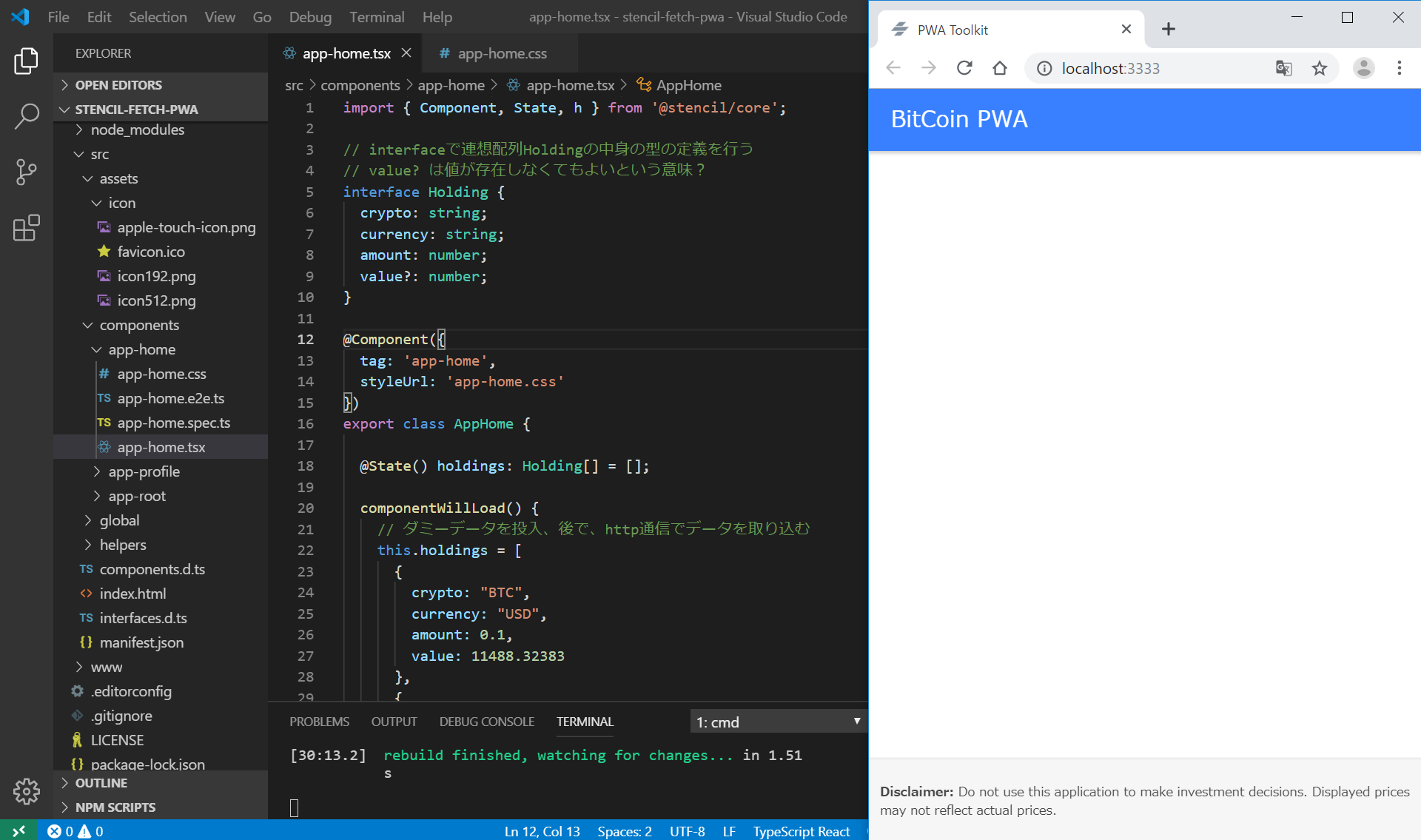
条件に応じて表示を変更する仕組み
this.nameが存在するときと存在しないときで表示を変更するような以下のコード
render() {
if (this.name) {
retugn ( <div>Hello {this.name}</div> )
} else {
return ( <div>Hello, World</div> )
}
}
これだと、render()の中身が膨大になりまくってしまうため、getName()関数を定義して、以下のように記載するとよいそうです。
render() {
return (
<div>Hello, {this.getName()}</div>
);
}
この方式で、holdings.lengthが無いときとあるときで、表示を変更するコードを、三項演算子(ハテナ?とセミコロン:)を用いて、以下のように記載します。
(参考:三項演算子(条件演算子) “A ? B : C”について)
renderWelcomeMessage() 関数
renderWelcomeMessage() {
return (
<div>
{!this.holdings.length ? (
<div class="message">
<p>
<strong>cryptoPWA</strong> is a <strong>P</strong>rogressive <strong>W</strong>eb{" "}
<strong>A</strong>pplication that allows you to keep track of the approximate worth of
your cryptocurency portfolio.
</p>
<p>
A PWA is like a normal application from the app store, but you can access it directly
through the web. You may also add this page to your home screen to launch it like your
other applications.
</p>
<p>
No account required, just hit the button below to start tracking your coins in
whatever currency you wish!
</p>
<ion-button href="/add-holding" color="primary">
Add Coins
</ion-button>
</div>
) : null}
</div>
);
}
//render()の中身の<ion-content>も以下のように変更
<ion-content>
{this.renderWelcomeMessage()}
<ion-list lines="none"></ion-list>
</ion-content>,
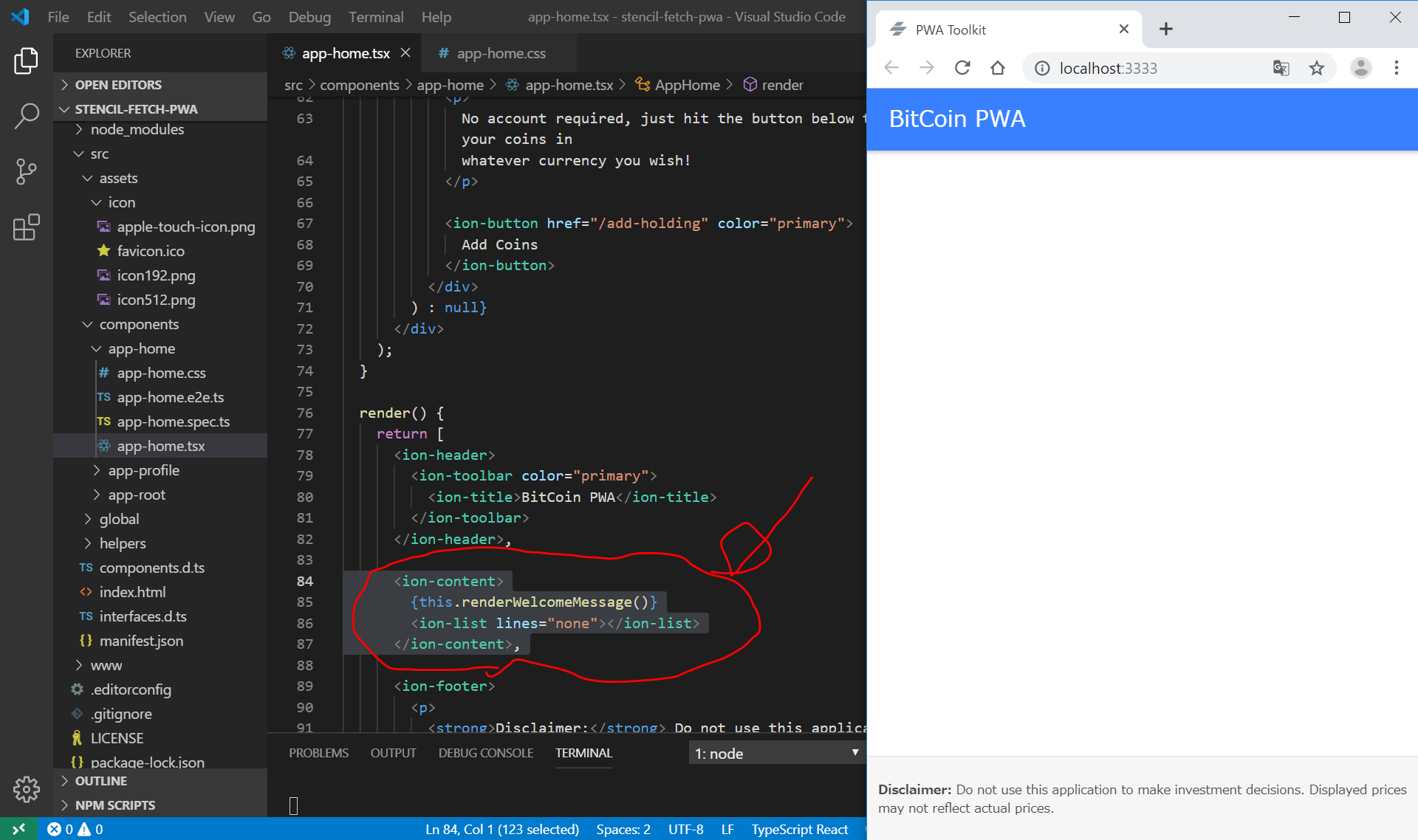
map関数を用いてデータを繰り返し表示する
今回、holdings[]配列の中身を順番に取り出して表示するコードをmap関数を用いて、<ion-content>の中身を以下のように変更します。
<ion-content>
{this.renderWelcomeMessage()}
<ion-list lines="none">
{this.holdings.map(holding => (
<ion-item-sliding>
<ion-item class="holding">
<ion-label>
<p>
<strong>
{holding.crypto}/{holding.currency}
</strong>
</p>
<p class="amount">
<strong>Coins:</strong> {holding.amount} <strong>Value:</strong> {holding.value}
</p>
<p class="value">{holding.amount * holding.value}</p>
</ion-label>
</ion-item>
<ion-item-options>
<ion-item-option color="danger">
<ion-icon slot="icon-only" name="trash"></ion-icon>
</ion-item-option>
</ion-item-options>
</ion-item-sliding>
))}
</ion-list>
</ion-content>,
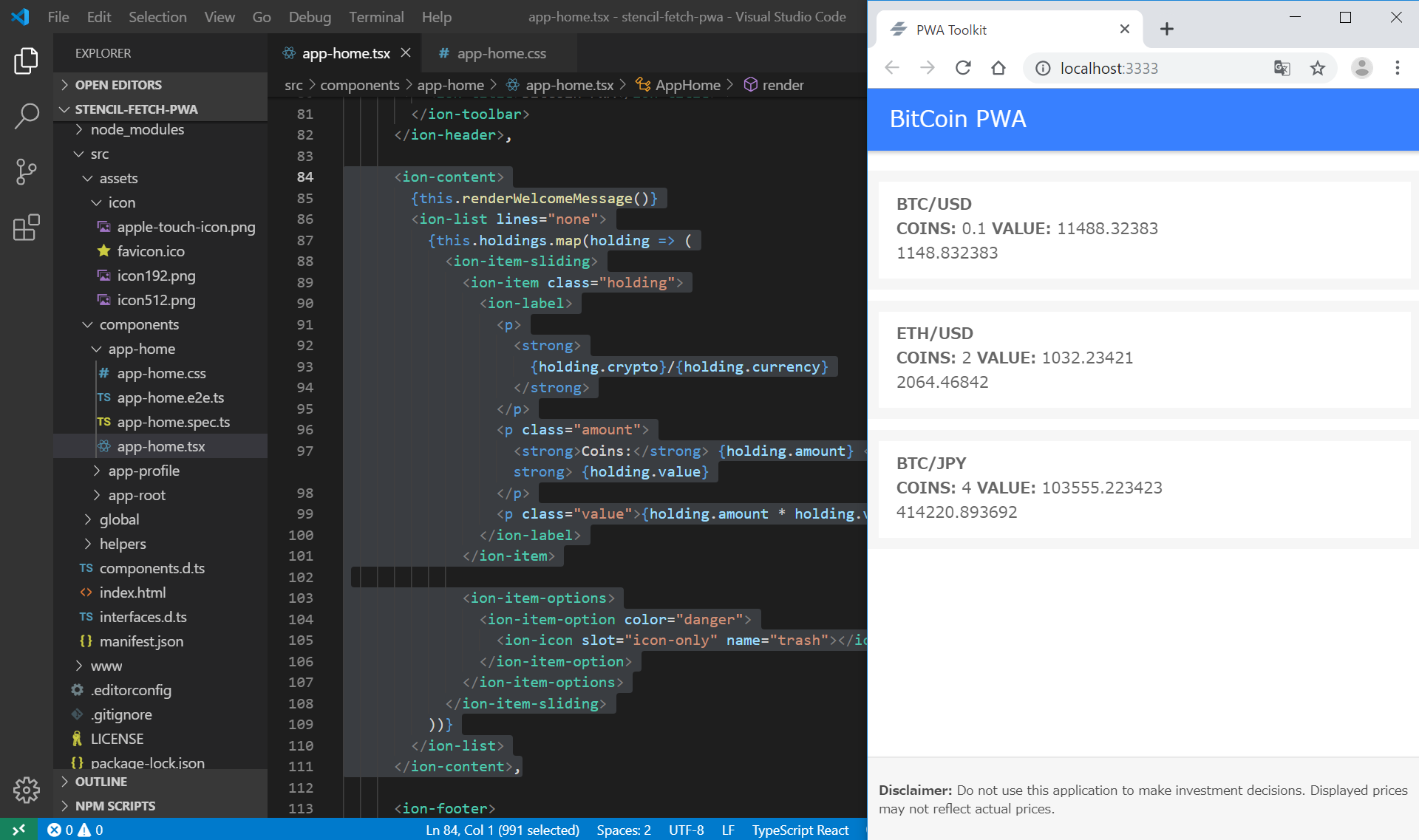
なんか、少しだけ、それっぽくなってきました。
次回は、引き続き、こちらのサイトを写経していきたいと思います。
ここまでのソースコード
https://github.com/adash333/stencil-fetch-pwa/tree/a97fa27ca1c7e0e5e3b696788b52ad591562d406
今回写経したサイト
https://www.joshmorony.com/building-a-pwa-with-stencil-rendering-layouts/
Building a PWA with Stencil: Rendering Layouts
BY JOSH MORONY | LAST UPDATED: SEPTEMBER 03, 2019
参考リンク
https://qiita.com/Leapin_JP/items/caed57ec30d638e40728
@Leapin_JP
2019年03月27日に更新
ブラウザレンダリング入門〜知ることで見える世界〜
Ionicの書き方については、公式ドキュメントを見る必要があります。
https://ionicframework.com/jp/docs/api/toolbar
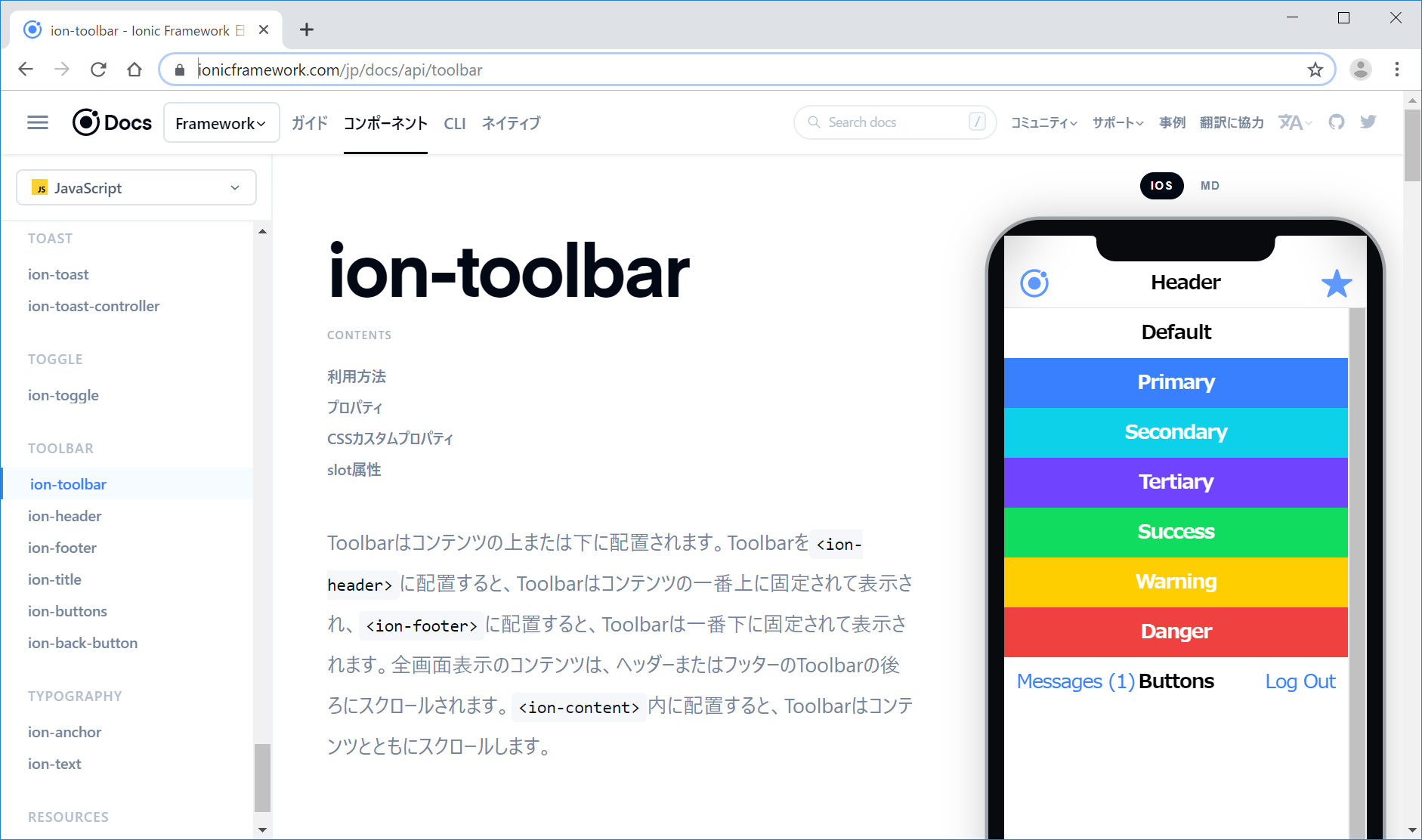
また、以下のサイトも参考にしていただければ幸いです。
Ionic3からIonic4への変更点
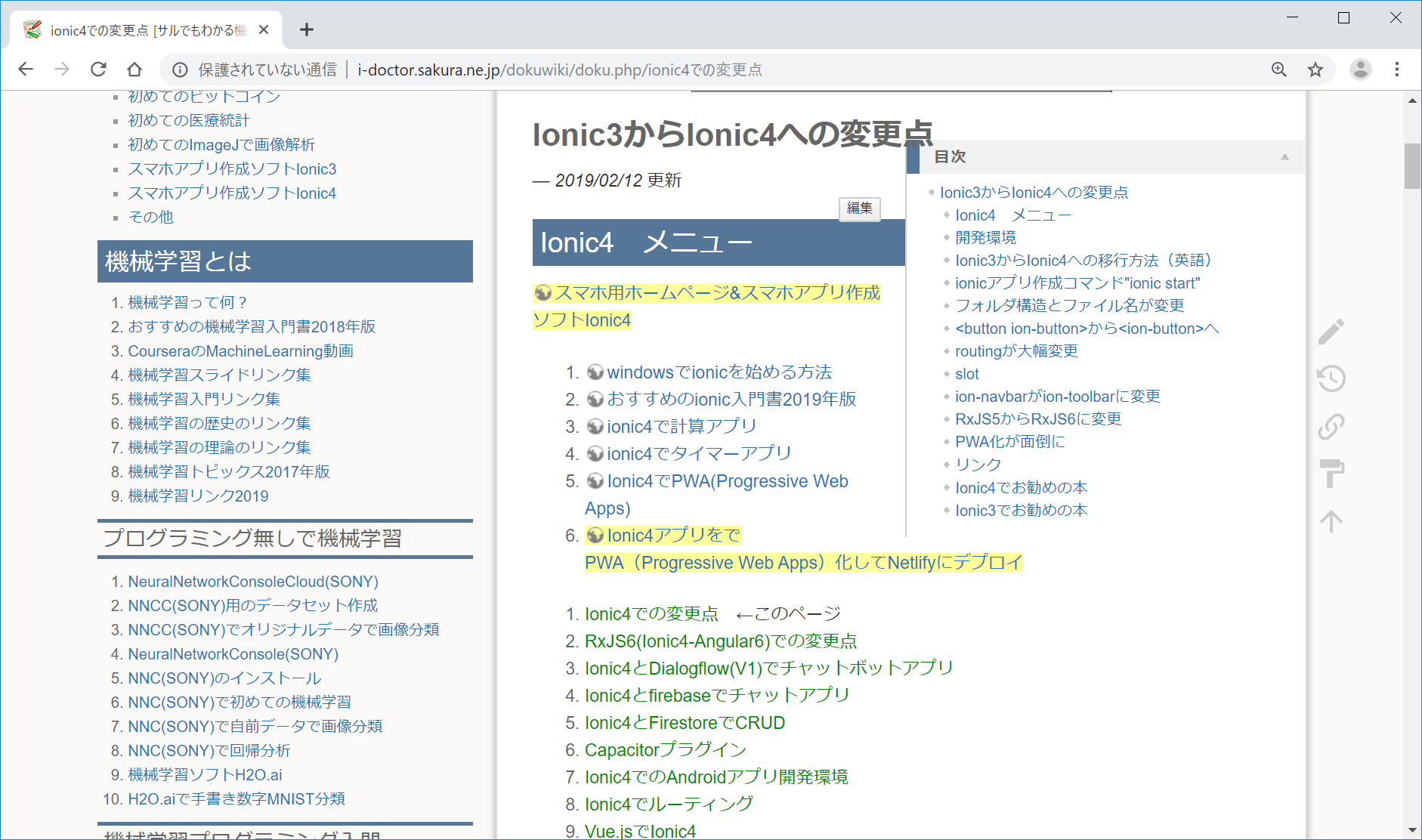
ディスカッション
コメント一覧
まだ、コメントがありません